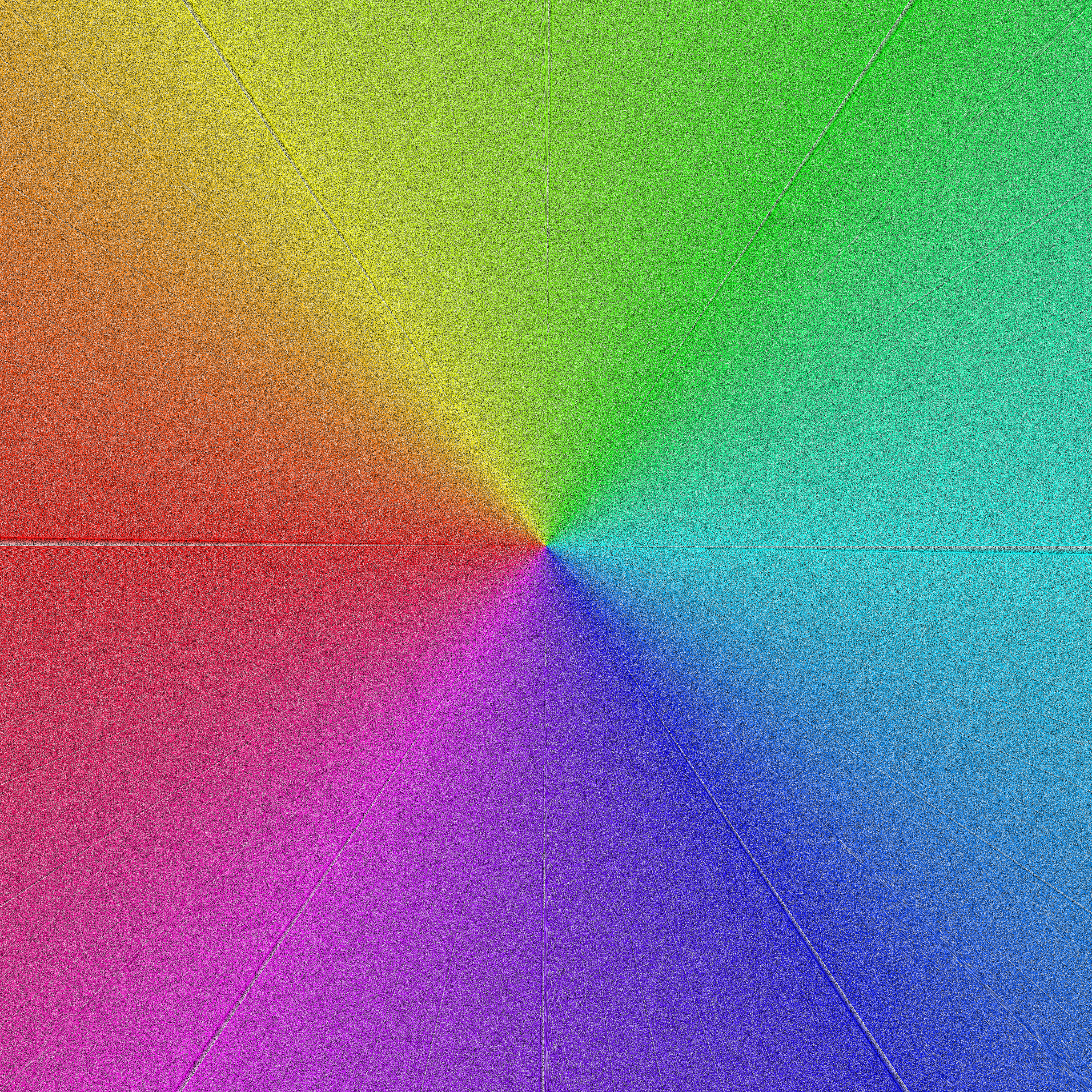
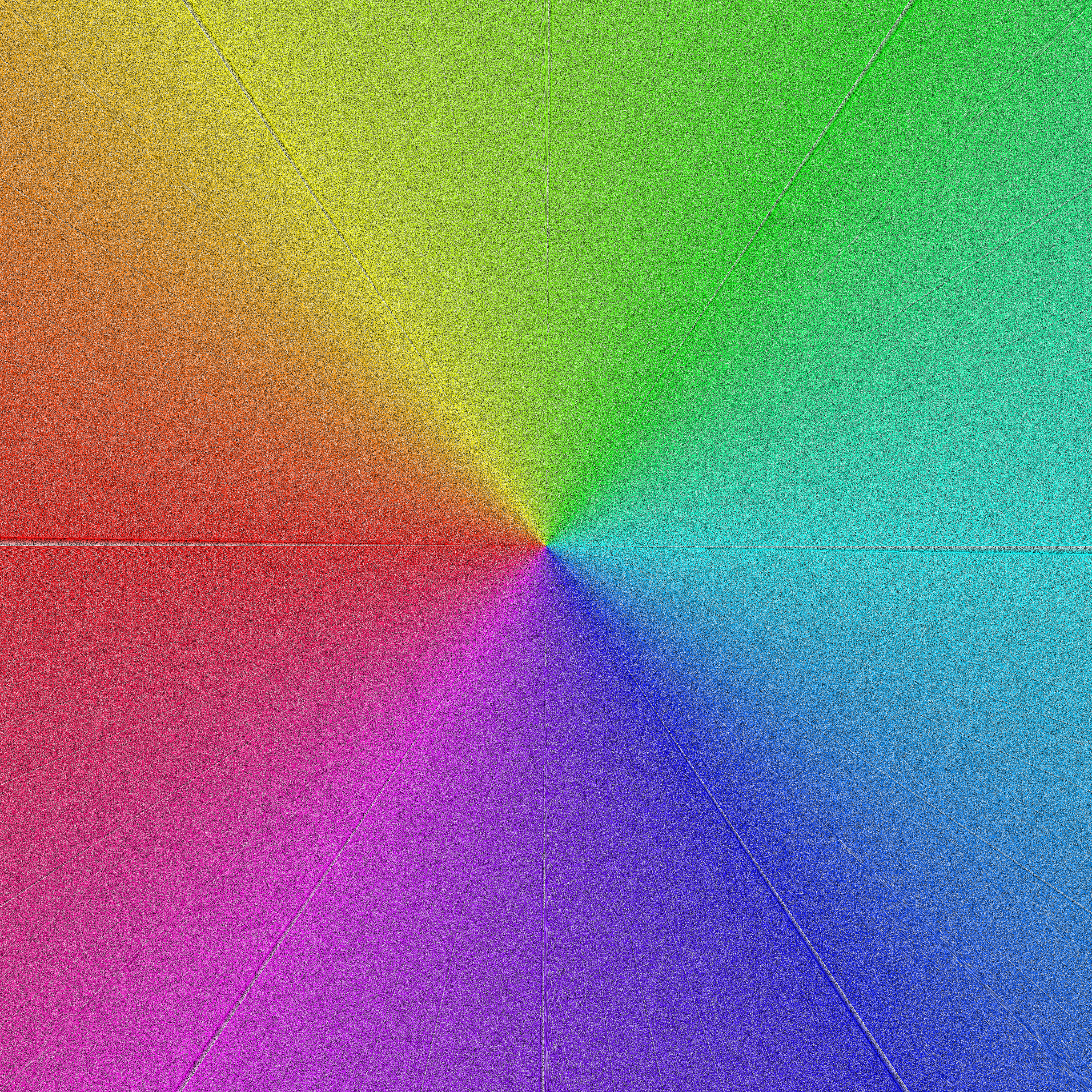
A little chat with ChatGPT 4o returned the following Python code, which I then ran in Google Colab:
import numpy as np from PIL import Image import colorsys # Image size: 4096 x 4096 = 16,777,216 pixels WIDTH = HEIGHT = 4096 CENTER_X, CENTER_Y = WIDTH // 2, HEIGHT // 2 # Generate all RGB colors rgb_colors = np.array([[r, g, b] for r in range(256) for g in range(256) for b in range(256)], dtype=np.uint8) # Convert RGB to HSB (HSV), normalized to 0–1 range def rgb_to_hsv(rgb): return np.array([colorsys.rgb_to_hsv(r/255, g/255, b/255) for r, g, b in rgb]) # Convert HSB (HSV) back to RGB 0–255 def hsv_to_rgb(hsv): return np.array([colorsys.hsv_to_rgb(h, s, v) for h, s, v in hsv]) * 255 # Convert all RGB to HSV to enable sorting hsv_colors = rgb_to_hsv(rgb_colors) # Assign image coordinates to each color using radial HSB mapping # Hue: angle around center # Saturation: distance from center # Brightness: vertical axis (top = bright, bottom = dark) coords = [] for y in range(HEIGHT): for x in range(WIDTH): dx = x - CENTER_X dy = y - CENTER_Y r = np.hypot(dx, dy) / (WIDTH / 2) # radius as saturation theta = (np.arctan2(dy, dx) + np.pi) / (2 * np.pi) # hue from angle (0–1) v = 1.0 - (y / HEIGHT) # brightness vertically coords.append((theta, r, v, x, y)) # Sort all RGB colors by their HSV mapping to the image layout # Then place each color exactly once coords.sort() # sorts by hue, then sat, then bright # Sort the HSV list the same way to match coordinates hsv_sorted_idx = np.lexsort((hsv_colors[:, 2], hsv_colors[:, 1], hsv_colors[:, 0])) rgb_sorted = rgb_colors[hsv_sorted_idx] # Create image image = Image.new("RGB", (WIDTH, HEIGHT)) pixels = image.load() for (h, s, v, x, y), color in zip(coords, rgb_sorted): pixels[x, y] = tuple(color) # Save image (lossless) image.save("radial_hsb_rgb_visualization.png", "PNG")
Date | |
---|---|
Colors | 16,777,216 |
Pixels | 16,777,216 |
Dimensions | 4,096 × 4,096 |
Bytes | 49,641,556 |