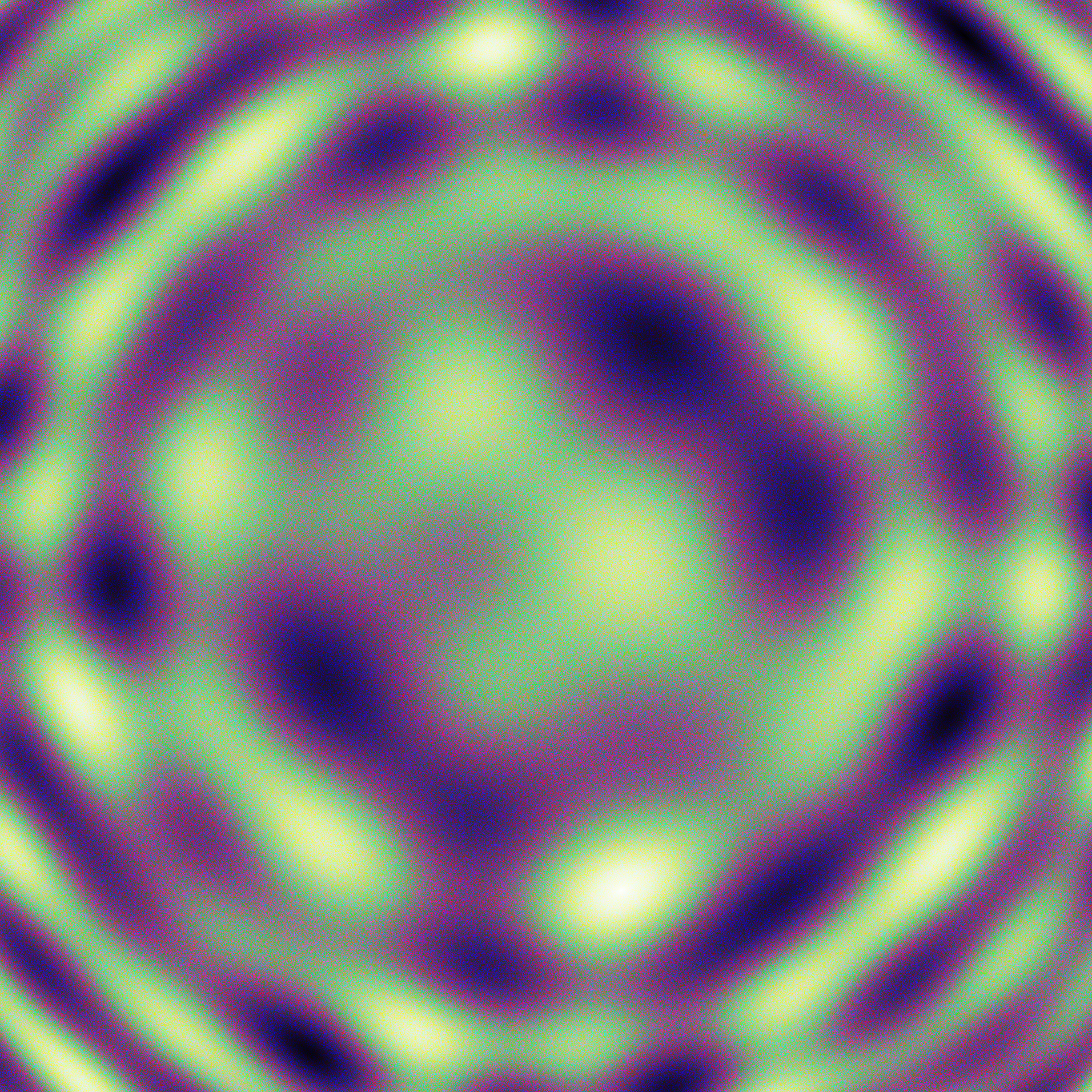
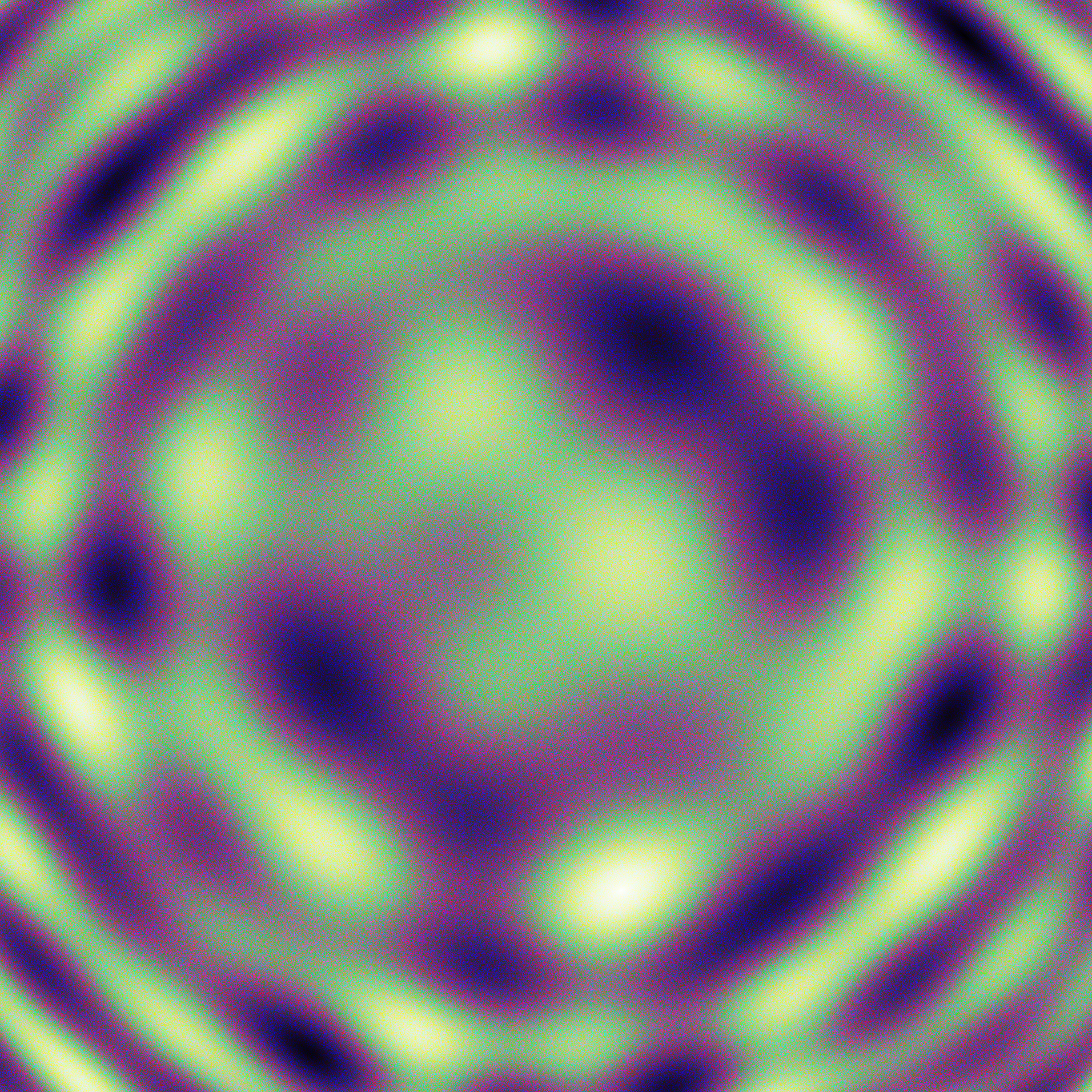
A little chat with ChatGPT 4o returned the following Python code, which I then ran in Google Colab:
import numpy as np from PIL import Image import colorsys # Image size WIDTH = HEIGHT = 4096 TOTAL_COLORS = WIDTH * HEIGHT # Step 1: Generate all RGB colors — exactly once colors = np.zeros((TOTAL_COLORS, 3), dtype=np.uint8) index = 0 for r in range(256): for g in range(256): for b in range(256): colors[index] = (r, g, b) index += 1 assert index == TOTAL_COLORS, "Color generation failed" # Step 2: Generate quantum-inspired coordinate map # Convert coordinates to "wave-interference energy" for sorting coords = [] for y in range(HEIGHT): for x in range(WIDTH): # Normalize to [-1, 1] nx = (x - WIDTH / 2) / (WIDTH / 2) ny = (y - HEIGHT / 2) / (HEIGHT / 2) # Simulate quantum field interference (wave-like energy) wave = ( np.sin(10 * nx) * np.cos(10 * ny) + np.sin(20 * nx * ny) + np.cos(15 * (nx**2 + ny**2)) ) coords.append((wave, x, y)) # Step 3: Sort pixels by wave function output coords.sort() # Step 4: Sort colors for aesthetic match (e.g., brightness) brightness = np.dot(colors, [0.299, 0.587, 0.114]) sorted_color_indices = np.argsort(brightness) colors_sorted = colors[sorted_color_indices] # Step 5: Create image and assign each color to its sorted coordinate img = Image.new("RGB", (WIDTH, HEIGHT)) pixels = img.load() for (i, (_, x, y)) in enumerate(coords): pixels[x, y] = tuple(colors_sorted[i]) # Step 6: Save image losslessly img.save("quantum_field_color_space.png", format="PNG")
Date | |
---|---|
Colors | 16,777,216 |
Pixels | 16,777,216 |
Dimensions | 4,096 × 4,096 |
Bytes | 50,206,691 |