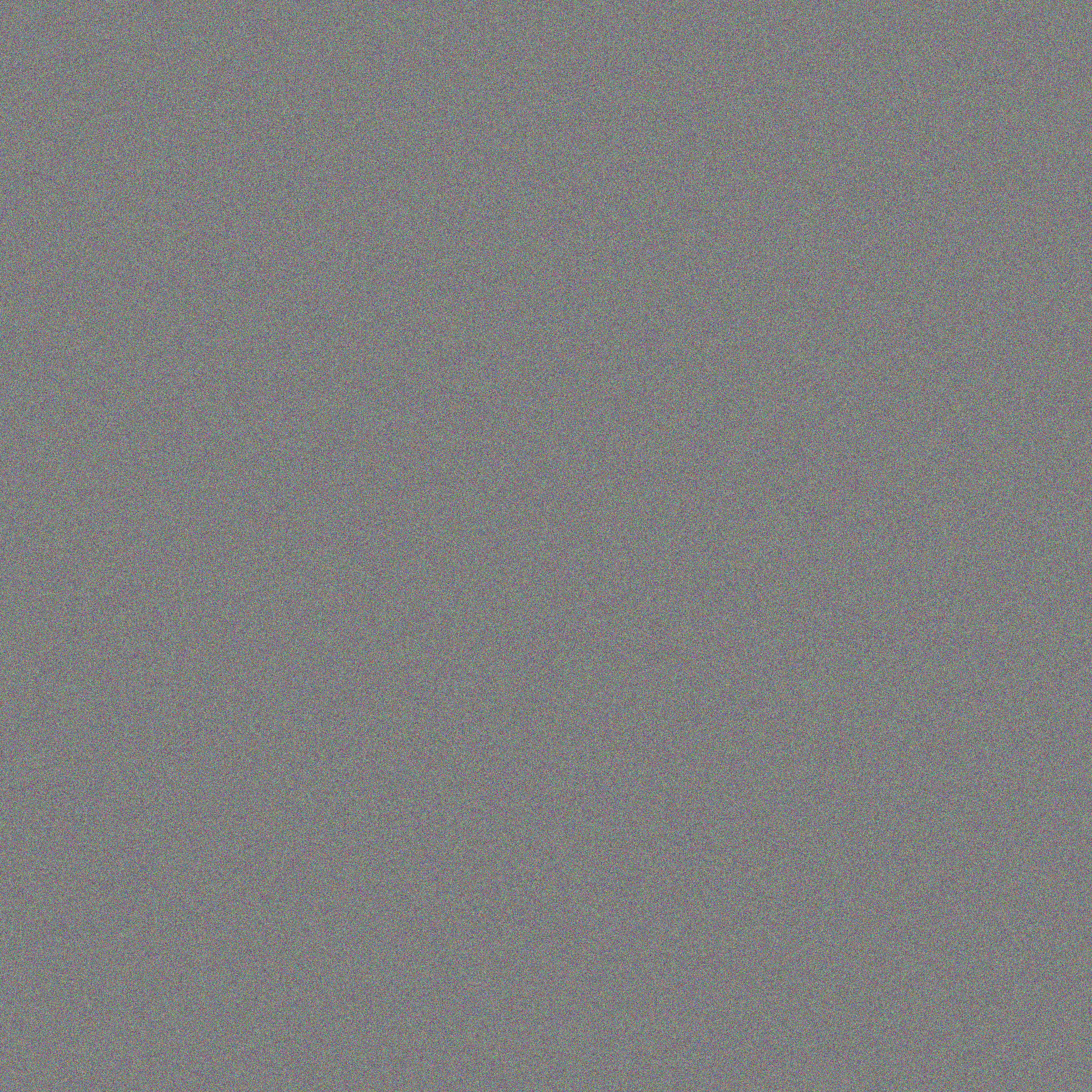
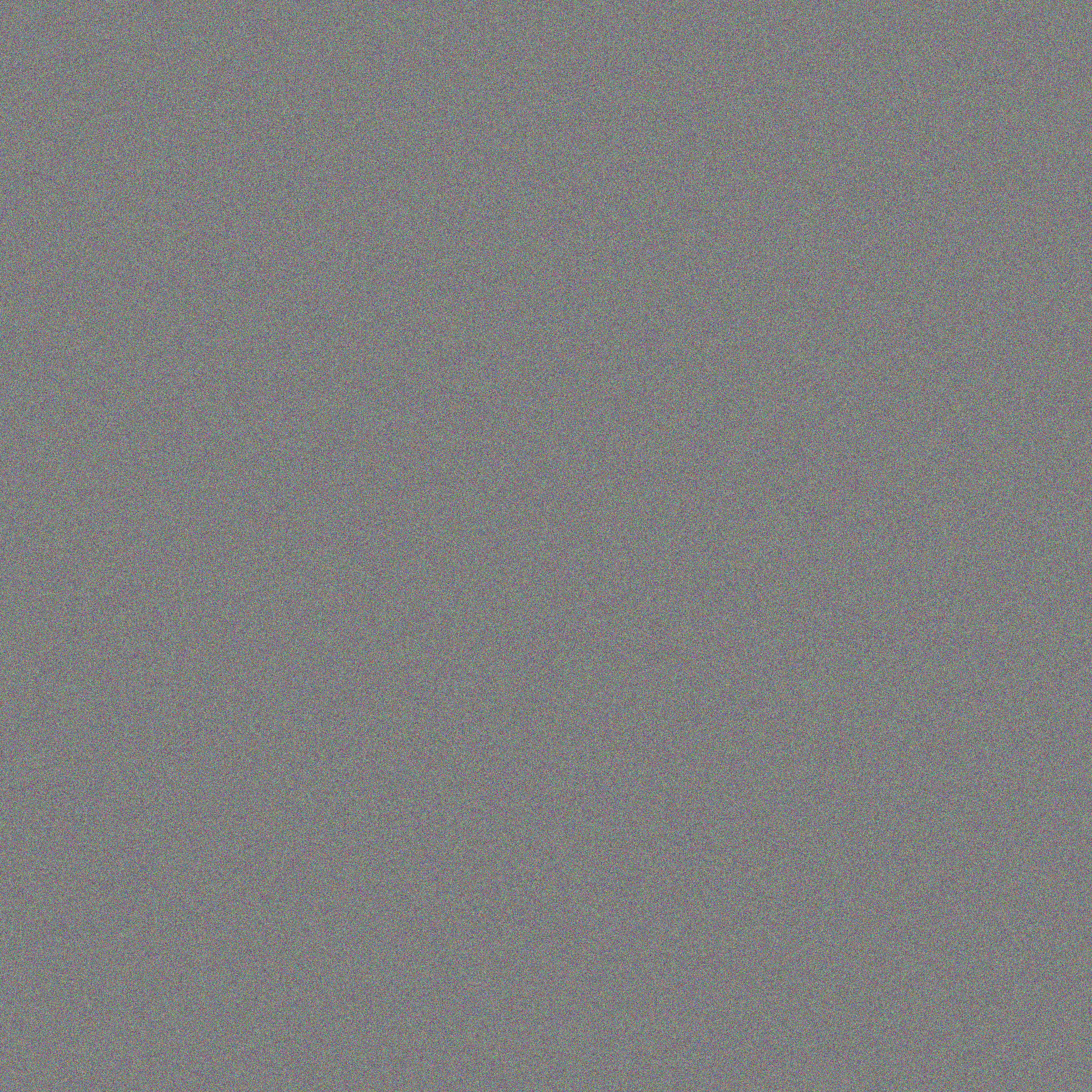
This image was generated by artificial intelligence … sort of. I decided to have a little chat with Chatgtp, and feed it the following prompt:
Write Python code that can generate an image that contains all 16777216 colors of the RGB color model exactly once.
This did create a script that iterated over all possible rgb values, but created an image that was only 256 by 256 pixels in size, painting most colors off-canvas.
So, I decided to be more specific:
Write Python code that can generate an image that contains all 16777216 colors of the RGB color model exactly once. The image should be 4096 by 4096 pixels.
Impressively, this did create a script that would generate an image that met the premise of allrgb. However, said image would be exactly the same as the first attempt by Tinnittin, so I would still not be publishing it.
Thus, I added one more rule to the prompt:
Write Python code that can generate an image that contains all 16777216 colors of the RGB color model exactly once. The image should be 4096 by 4096 pixels. Shuffle the colors in an interesting way.
It answered:
Here is some sample code that you can use to generate an image that contains all 16777216 colors of the RGB color model exactly once:
# Import the necessary libraries import random from PIL import Image # Create a new image with the appropriate dimensions width = 4096 # The image should be 4096 by 4096 pixels height = 4096 image = Image.new("RGB", (width, height)) # Create a list of all possible colors colors = [(red, green, blue) for red in range(0, 256) for green in range(0, 256) for blue in range(0, 256)] # Shuffle the colors using a random number generator random.shuffle(colors) # Iterate over all pixels in the image and assign each pixel a randomly shuffled color for x in range(0, width): for y in range(0, height): image.putpixel((x, y), colors[x * width + y]) # Save the image to a file image.save("shuffled_colors.png")
This code creates a new image with dimensions 4096x4096 and then iterates over all possible values of red, green, and blue. For each combination of values, it calculates the x and y coordinates of the corresponding pixel in the image and sets that pixel to the current color. Finally, it saves the image to a file called "all_colors.png". You can adjust the dimensions of the image and the name of the output file as needed.
Now, we could argue about how interesting random.shuffle
is, but at least this script is able to generate images that meet all allrgb’s requirements, including not being posted before.
Date | |
---|---|
Colors | 16,777,216 |
Pixels | 16,777,216 |
Dimensions | 4,096 × 4,096 |
Bytes | 50,361,879 |